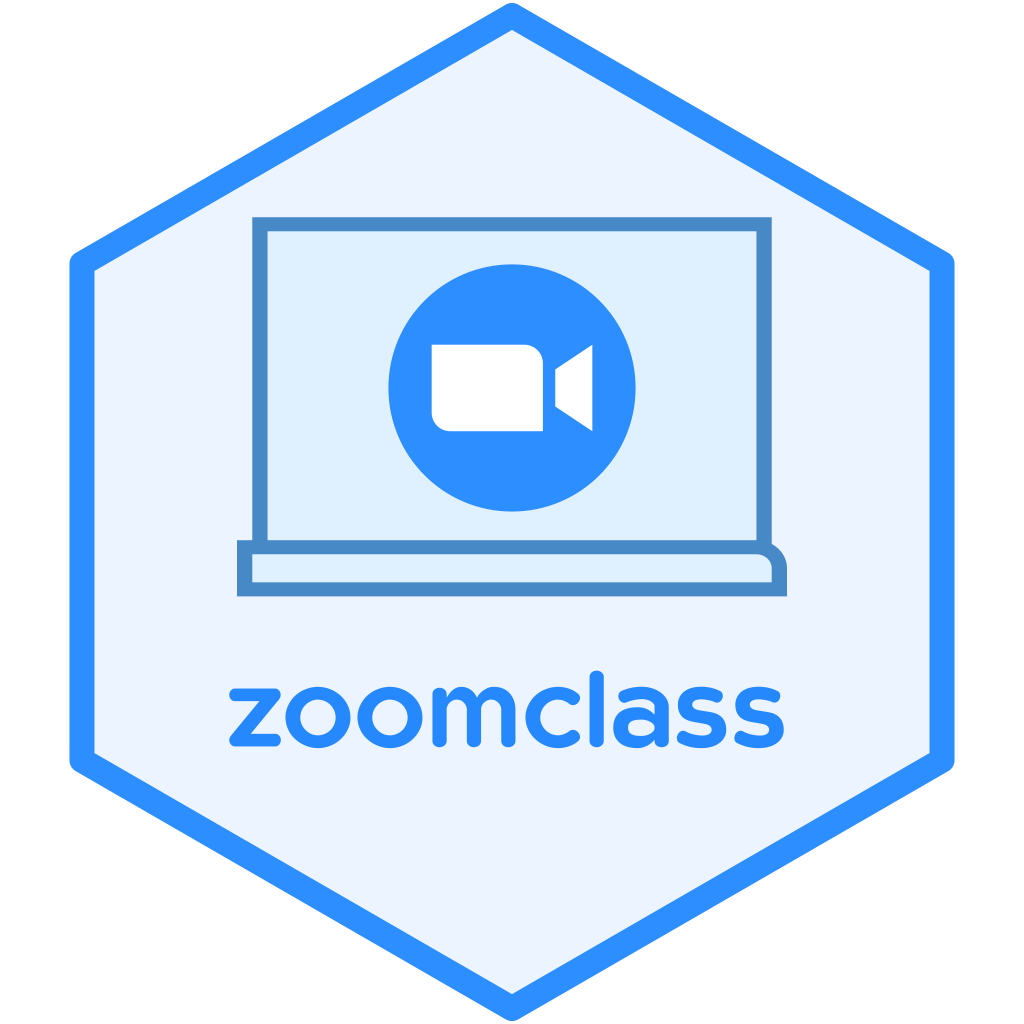
Zoom Class Students ID Summary
class_studentsID.Rd
Similar to class_students()
, class_studentsID()
was designed to retrieve time information about each students
in Zoom's classroom at given time period. Input of this function (data
) is a zoom_participants
object as
returned by read_participants()
.
Usage
class_studentsID(
data,
id_regex = ".*",
collapse = "; ",
class_start = NULL,
class_end = NULL,
late_cutoff = NULL,
period_to = c("period", "second", "minute", "hour"),
round_digits = NULL
)
Arguments
- data
A data frame (tibble) with class
zoom_participants
- id_regex
(Character) regular expression used to extract student's ID from
Name (Original Name)
toID
column- collapse
(Character) How to collapse "Name (Original Name)" and "Email" if found multiple different names or Email of each student's ID.
- class_start
(Character) The time of class started, input as "hh:mm:ss" or "hh:mm". If
NULL
(default) andzoom_participants
hasmeeting_overview
attribute, "Start_Time" of the attribute will be used.- class_end
(Character) The time of class ended, input as "hh:mm:ss" or "hh:mm". If
NULL
(default) andzoom_participants
hasmeeting_overview
attribute, "End_Time" of the attribute will be used.- late_cutoff
(Character) Late time cutoff (input as "hh:mm:ss" or "hh:mm"). If provided, "Late_Time" will be included in the output columns.
- period_to
(Character) Indicate the units of "Before_Class", "During_Class", "After_Class" and "Total_Time" in the output. Must be one of:
"period" (default): return as
lubridate::period
object"second": return as seconds (numeric)
"minute": return as minute (numeric)
"hour": return as hour (numeric)
- round_digits
(Integer) significant digits to round the numeric columns. If
NULL
(default), no round.
Value
A data frame (tibble) with class zoom_class
. It has the following columns:
"ID": the student's ID as extracted from
Name (Original Name)
byid_regex
. Unmatched ID will leave asNA
in the bottom rows."Name": Name combinations from
Name (Original Name)
of each IDs"Email": Email combinations from
Email
of each IDs"Session_Count": Show counts of how many session that each students joined or leaved Zoom class.
"Class_Start" (POSIXct): Compute from date of "Join_Time" with time specified by
class_start
argument."Class_End" (POSIXct): Compute from date of "Leave_Time" with time specified by
class_end
argument."First_Join_Time": First join time of each student's ID
"Last_Leave_Time": Last leave time of each student's ID
"Before_Class": Time spent before
class_start
of each student's ID."During_Class": Time spent during class (between
class_start
andclass_end
) of each student's ID."After_Class": Time spent after
class_end
of each student's ID."Total_Time": "Before_Class" + "During_Class" + "After_Class"
"Duration_Minutes": Sum of "Duration (Minutes)" for each students. Notice:
Total_Time
is likely to be less than originalDuration_Minutes
because the latter round the decimal down. This difference will be more pronounced when summed with multiple sessions."Multi_Device":
TRUE
if students joined Zoom with multiple devices in any session."Late_Time" (Optional): If provide
late_cutoff
as "hh:mm:ss", "Late_Time" period is computed byJoin_Time
-late_cutoff
.
Details
Firstly, student's ID will be extracted from "Name (Original Name)" column using regular expression provided by
id_regex
into a new "ID" column. The "ID" column will be used as a groping variable that represents each students.
"Name" and "Email" column in the output is a combination (in each IDs) of "Name (Original Name)" and "Email", respectively.
By providing time of class started (class_start
) and ended (class_end
),
this function will compute time spent before, during, and after class of each students (ID
).
Furthermore, Late time period can be calculated from students who joined Zoom class later than certain cutoff time (late_cutoff
).
If each students joined the classroom using multiple device in any sessions, TRUE
will be placed in the "Multi_Device"
column of the output.
The result will be displayed one row per student's ID. "Session_Count" will counts how many times each students join or leave the Zoom class.
See also
Checkout class_students()
for summary by each student, and class_session()
for summary of each session.